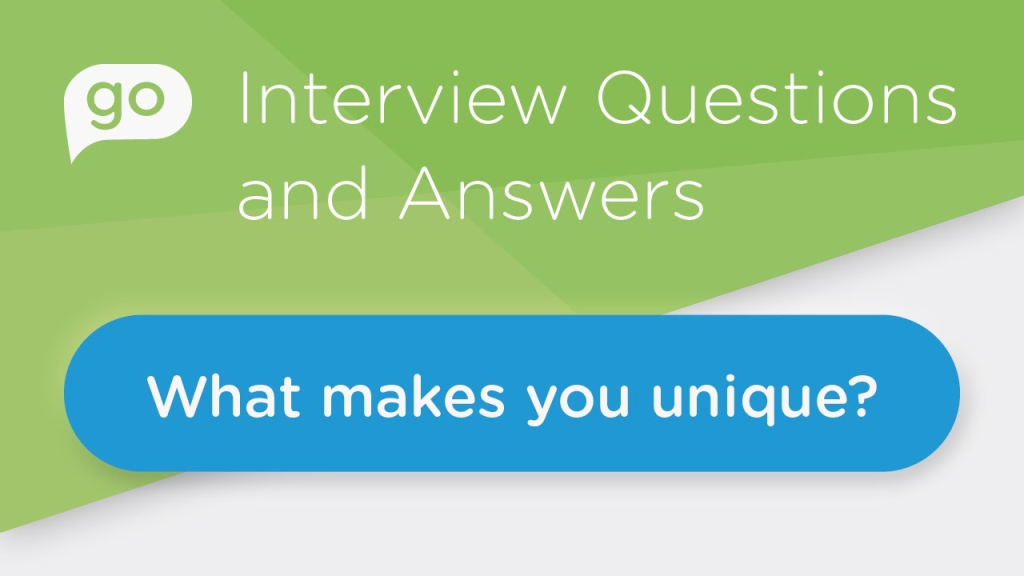
1) Which of the following is a valid reason to use Goroutines?
a) To achieve parallelism
b) To achieve concurrency
c) To implement containerization
d) None of the above
Answer: a
2) What is the defer statement used for?
a) To postpone the execution of a statement until the surrounding function returns
b) To prevent race conditions in parallel programs
c) To handle errors in a more efficient way
d) None of the above
Answer: a
3) Which data types in Go are considered composite?
a) struct and array
b) map and slice
c) interface and channel
d) All of the above
Answer: b
4) What is the difference between a slice and an array?
a) An array is fixed size, while a slice is dynamic
b) A slice is fixed size, while an array is dynamic
c) An array is a type, while a slice is a data structure
d) None of the above
Answer: a
5) What is the purpose of channels in Go?
a) To synchronize Goroutines
b) To pass values between Goroutines
c) To handle errors in parallel programs
d) None of the above
Answer: b
6) Which of the following is a valid method for preventing race conditions in Go?
a) Using mutexes
b) Using channels
c) Using Goroutines
d) All of the above
Answer: a
7) Which of the following is a valid way to create a slice in Go?
a) s := []int{1, 2, 3}
b) s := make([]int, 3)
c) s := [3]int{1, 2, 3}
d) All of the above
Answer: d
8) Which of the following is the correct syntax for a Goroutine?
a) go myFunction()
b) goroutine myFunction()
c) thread myFunction()
d) None of the above
Answer: a
9) What is a pointer in Go?
a) A variable that stores the address of another variable
b) A special type of function
c) A type of loop
d) None of the above
Answer: a
10) Which of the following is a valid way to declare a map in Go?
a) m := make(map[int]int)
b) m := map[int]int{}
c) m := map[int]int{1: 2, 3: 4}
d) All of the above
Answer: d
11) Which of the following is a valid way to declare a struct in Go?
a) type myStruct struct{}
b) type myStruct struct {name string; age int}
c) type myStruct {name string; age int}
d) All of the above
Answer: d
12) What is a closure in Go?
a) A function that does not have a name
b) A function that returns a function
c) A function that closes a channel
d) None of the above
Answer: b
13) What is the role of the init() function in a package?
a) To initialize global variables
b) To provide a main function for the package
c) To handle dependencies between packages
d) None of the above
Answer: a
14) Which of the following is a valid way to create a new error in Go?
a) errors.New(“my error”)
b) panic(“my error”)
c) fmt.Errorf(“my error”)
d) All of the above
Answer: a
15) What is the purpose of the select statement in Go?
a) To allow a Goroutine to listen for incoming data on multiple channels
b) To handle signals from the operating system
c) To select a Goroutine for execution
d) None of the above
Answer: a
16) What is a Goroutine leak in Go?
a) A situation where a Goroutine is blocked indefinitely
b) A situation where a Goroutine is not properly terminated
c) A situation where a Goroutine is consuming too much memory
d) None of the above
Answer: b
17) Which of the following is a valid way to create a new Goroutine?
a) go myFunction()
b) myFunction()
c) createGoroutine(myFunction())
d) None of the above
Answer: a
18) What is the correct syntax for a switch statement in Go?
a) switch x { case 1: break }
b) switch { case x == 1: break }
c) switch x { case == 1: break }
d) None of the above
Answer: b
19) What is the difference between the ++ and — operators in Go and other programming languages?
a) There is no difference
b) In Go, the ++ and — operators can only be used as postfix operators
c) In Go, the ++ and — operators have a side effect of returning the old value
d) None of the above
Answer: c
20) Which of the following is a valid way to declare an interface in Go?
a) type myInterface interface{}
b) type myInterface interface {myFunction() int}
c) type myInterface {myFunction() int}
d) All of the above
Answer: b
21) What is the difference between the append and copy functions in Go?
a) Append adds elements to a slice, while copy replaces elements in a slice
b) Copy adds elements to a slice, while append replaces elements in a slice
c) Append and copy are the same function with different names
d) None of the above
Answer: a
22) Which of the following is a valid way to declare a constant in Go?
a) const myConstant = “hello”
b) const myConstant string = “hello”
c) var myConstant = “hello”
d) None of the above
Answer: a
23) What is the purpose of the range keyword in Go?
a) To iterate over the elements of an array, slice, or map
b) To declare a loop variable
c) To compare two variables for equality
d) None of the above
Answer: a
24) What is the difference between nil and a zero value in Go?
a) Nil is the zero value of a pointer, while a zero value is the default value for other types
b) Nil is the zero value for all types in Go
c) Nil is an invalid value, while a zero value is a valid value
d) None of the above
Answer: a
25) What is the purpose of the panic() function in Go?
a) To handle errors in a more efficient way
b) To stop the execution of a program immediately
c) To create a new Goroutine
d) None of the above
Answer: b
26) Which of the following is a valid way to sort a slice of integers in Go?
a) sort(s)
b) sort.Ints(s)
c) s.Sort()
d) None of the above
Answer: b
27) What is the difference between a value receiver and a pointer receiver in Go?
a) A value receiver copies the value, while a pointer receiver modifies the original value
b) A value receiver modifies the original value, while a pointer receiver copies the value
c) A value receiver is used for immutable types, while a pointer receiver is used for mutable types
d) None of the above
Answer: a
28) Which of the following is a valid way to read data from a channel in Go?
a) val <- chan
b) chan <- val
c) <- val
d) None of the above
Answer: a
29) What is the purpose of the sync package in Go?
a) To provide concurrency-safe data structures
b) To provide synchronization primitives like mutexes and condition variables
c) To provide tools for profiling and debugging concurrent programs
d) All of the above
Answer: d
30) Which of the following is a valid way to use the log package in Go?
a) log(“message”)
b) log.Println(“message”)
c) fmt.Println(“message”)
d) None of the above
Answer: b
31) What is the zero value of a boolean variable in Go?
a) true
b) false
c) nil
d) None of the above
Answer: b
32) Which of the following is a valid way to create an anonymous function in Go?
a) func(int, int) int {}
b) lambda int, int { return int + int }
c) func(x, y int) int { return x + y }
d) None of the above
Answer: c
33) Which of the following is a valid way to declare a constant array in Go?
a) const arr = [3]int{1, 2, 3}
b) const arr [3]int{1, 2, 3}
c) const arr []int{1, 2, 3}
d) None of the above
Answer: a
34) Which of the following is a valid way to write a string literal in Go?
a) “hello”
b) ‘hello’
c) hello
d) None of the above
Answer: a
35) Which of the following is a valid way to open a file in Go?
a) f, err := os.Open(“file.txt”)
b) f := open(“file.txt”)
c) f, err := open(“file.txt”)
d) None of the above
Answer: a
36) Which of the following is a valid way to use the fmt package in Go?
a) fmt(“message”)
b) fmt.Println(“message”)
c) print(“message”)
d) None of the above
Answer: b
37) What is the purpose of the break statement in Go?
a) To exit a loop immediately
b) To return a value from a function
c) To switch to another Goroutine
d) None of the above
Answer: a
38) Which of the following is a valid way to declare a pointer to a struct in Go?
a) var p myStruct = new(myStruct)
b) var p myStruct = &myStruct{}
c) var p myStruct = new(myStruct)
d) None of the abov
Answer: a
39) What is the purpose of the len() function in Go?
a) To return the length of a slice, array, or map
b) To convert a string to an integer
c) To calculate the logarithm of a number
d) None of the above
Answer: a
40) Which of the following is a valid way to defer a function call in Go?
a) defer myFunction()
b) go myFunction()
c) myFunction.Defer()
d) None of the above
Answer: a
41) What is the purpose of the time package in Go?
a) To provide tools for measuring time and handling time-related operations
b) To provide tools for profiling and debugging concurrent programs
c) To provide synchronization primitives like mutexes and condition variables
d) None of the above
Answer: a
42) Which of the following is a valid way to write a comment in Go?
a) / This is a comment
b) // This is a comment
c) # This is a comment
d) None of the above
Answer: b
43) What is the difference between log.Fatal() and log.Panic() in Go?
a) There is no difference
b) log.Fatal() terminates the program, while log.Panic() does not
c) log.Panic() terminates the program, while log.Fatal() does not
d) None of the above
Answer: b
44) Which of the following is a valid way to create a new channel in Go?
a) chan myChannel
b) make(chan myChannel)
c) make(chan myChannel, bufferLength)
d) None of the above
Answer: c
45) What is the purpose of the recover() function in Go?
a) To handle the deferred execution of a function
b) To recover from a panic()
c) To handle a signal from the operating system
d) None of the above
Answer: b
46) Which of the following is a valid way to use the net package in Go?
a) net.Dial(“tcp”, “localhost:8000”)
b) net.Open(“tcp”, “localhost:8000”)
c) net.Listen(“tcp”, “localhost:8000”)
d) None of the above
Answer: a
47) What is the purpose of the context package in Go?
a) To provide tools for measuring time and handling time-related operations
b) To provide tools for profiling and debugging concurrent programs
c) To provide a way to pass context information between Goroutines
d) None of the above
Answer: c
48) Which of the following is a valid way to use the bufio package in Go?
a) bufio(“message”)
b) bufio.NewWriter(“file.txt”)
c) bufio.NewReader(f)
d) None of the above
Answer: c
49) What is the purpose of the os package in Go?
a) To provide tools for measuring time and handling time-related operations
b) To provide tools for working with the operating system
c) To provide synchronization primitives like mutexes and condition variables
d) None of the above
Answer: b
50) Which of the following is a valid way to use the json package in Go?
a) json.Parse(“data.json”)
b) json.Unmarshal([]byte(data), &myStruct)
c) json.Marshal(myStruct)
d) None of the above
Answer: b
- Top 10 DevOps Trainers in the world - October 7, 2023
- What is Cookies and Why it is Used? - May 24, 2023
- TOP trends of transitions in TikTok - May 10, 2023