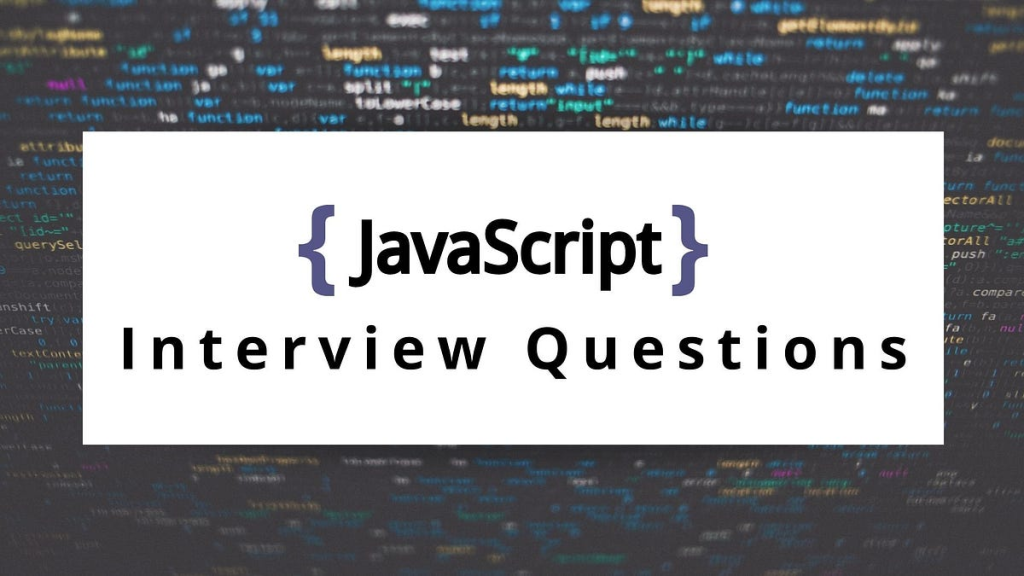
- What is JavaScript?
A. A programming language
B. A markup language
C. A web browser
Answer: A
- What is the output of console.log(“Hello” + 4)?
A. Hello4
B. TypeError
C. Hello
Answer: A
- Which one of the following is considered a falsy value in JavaScript?
A. null
B. NaN
C. undefined
Answer: A
- What is the difference between == and ===?
A. Both are the same
B. == only compares values, while === compares both values and data types
C. === only compares values, while == compares both values and data types
Answer: B
- What is an example of a higher-order function in JavaScript?
A. setTimeout()
B. console.log()
C. Math.random()
Answer: A
- What is the output of console.log(5 == “5”)?
A. true
B. false
C. undefined
Answer: A
- What is the output of console.log(typeof null)?
A. null
B. object
C. undefined
Answer: B
- What is the output of console.log([1, 2, 3] + [4, 5, 6])?
A. [1, 2, 3, 4, 5, 6]
B. 123456
C. NaN
Answer: B
- Which one of the following is an example of a JavaScript event?
A. click
B. div
C. span
Answer: A
- What is the output of console.log(+”5″)?
A. 5
B. “5”
C. TypeError
Answer: A
- What is the output of console.log(10%3)?
A. 1
B. 3
C. 0
Answer: A
- Which one of the following is a valid JavaScript variable name?
A. my-variable
B. my variable
C. myVariable
Answer: C
- What is the output of console.log(!”Hello”)?
A. false
B. true
C. “” (empty string)
Answer: B
- What is the output of console.log(5 + “5”)?
A. “55”
B. 10
C. TypeError
Answer: A
- What is an example of a JavaScript closure?
A. function add(x, y) { return x + y; }
B. function() { var x = 5; return function() { return x; } }
C. var x = 5; x++;
Answer: B
- What is the output of console.log(typeof undefined)?
A. undefined
B. null
C. object
Answer: A
- What is the output of console.log(Infinity – Infinity)?
A. NaN
B. Infinity
C. -Infinity
Answer: NaN
- What is the output of console.log(Math.floor(4.5))?
A. 4
B. 5
C. 4.5
Answer: A
- What is an example of a JavaScript constructor function?
A. function add(x, y) { return x + y; }
B. var obj = { name: “John”, age: 30 };
C. function Person(name, age) { this.name = name; this.age = age; }
Answer: C
- What is the output of console.log(Array.isArray({}))?
A. true
B. false
C. TypeError
Answer: B
- What is an example of a JavaScript callback function?
A. function add(x, y) { return x + y; }
B. setTimeout(function() { console.log(“Hello”); }, 1000);
C. var x = 5; x++;
Answer: B
- What is the output of console.log(Math.max([1, 2, 3]))?
A. 1
B. 2
C. 3
Answer: NaN
- What is an example of a JavaScript promise?
A. function add(x, y) { return x + y; }
B. new Promise(function(resolve, reject) { setTimeout(function() { resolve(“Hello”); }, 1000); });
C. var x = 5; x++;
Answer: B
- What is the output of console.log([“a”, “b”, “c”].splice(1, 1))?
A. [“a”, “c”]
B. [“b”, “c”]
C. “b”
Answer: B
- What is the output of console.log(“Hello John”.split(” “))?
A. “Hello John”
B. [“Hello”, “John”]
C. TypeError
Answer: B
- What is the output of console.log(20 + 30 + “40”)?
A. “2050”
B. “50”
C. TypeError
Answer: A
- What is the output of console.log(“Hello”.charAt(1))?
A. “h”
B. “e”
C. “l”
Answer: B
- What is an example of a JavaScript regular expression?
A. /hello/
B. function add(x, y) { return x + y; }
C. var x = 5; x++;
Answer: A
- What is the output of console.log(“Hello”.toUpperCase())?
A. “hello”
B. “HELLO”
C. TypeError
Answer: B
- What is the output of console.log([].constructor === Array)?
A. true
B. false
C. TypeError
Answer: A
- What is the output of console.log(“Hello”.replace(“l”, “x”))?
A. “Hexlo”
B. “Hxllo”
C. “Hello”
Answer: B
- What is the output of console.log(Math.ceil(4.1))?
A. 4
B. 5
C. 4.1
Answer: B
- What is an example of a JavaScript arrow function?
A. function add(x, y) { return x + y; }
B. var multiply = (x, y) => x * y;
C. var x = 5; x++;
Answer: B
- What is the output of console.log([1, 2, 3].map(x => x * 2))?
A. [2, 4, 6]
B. [1, 2, 3]
C. [4, 8, 12]
Answer: C
- What is the output of console.log(9 < 8 < 7)?
A. true
B. false
C. TypeError
Answer: A
- What is the output of console.log(“Hello”.length)?
A. 5
B. “Hello”
C. TypeError
Answer: A
- What is an example of a JavaScript IIFE (Immediately Invoked Function Expression)?
A. (function add(x, y) { return x + y; })(3, 4);
B. function() { var x = 5; return function() { return x; } }
C. function Person(name, age) { this.name = name; this.age = age; }
Answer: A
- What is the output of console.log(Math.round(4.4))?
A. 4
B. 5
C. 4.4
Answer: A
- What is the output of console.log(Math.random() * 10)?
A. A random number between 0 and 1
B. A random number between 0 and 10
C. A random number between 1 and 10
Answer: B
- What is the output of console.log([1, 2, 3].indexOf(2))?
A. 0
B. 1
C. 2
Answer: B
- What is the output of console.log(+”5″ + 5)?
A. 10
B. “10”
C. TypeError
Answer: A
- What is the output of console.log([“a”, “b”, “c”].indexOf(“d”))?
A. -1
B. 0
C. 1
Answer: A
- What is the output of console.log(“apple”.indexOf(“p”))?
A. 0
B. 1
C. 2
Answer: 1
- What is the output of console.log(“apple”.slice(1, 3))?
A. “ap”
B. “pp”
C. “pl”
Answer: C
- What is the output of console.log(“Hello”.substring(1, 3))?
A. “el”
B. “llo”
C. “He”
Answer: A
- What is the output of console.log([1, 2, 3].push(4))?
A. [1, 2, 3, 4]
B. [4, 3, 2, 1]
C. 4
Answer: C
- What is an example of a JavaScript generator function?
A. function add(x, y) { return x + y; }
B. function* generate() { yield 1; yield 2; yield 3; }
C. var x = 5; x++;
Answer: B
- What is the output of console.log(Math.min([1, 2, 3]))?
A. 1
B. 2
C. 3
Answer: NaN
- What is an example of a JavaScript async/await function?
A. function add(x, y) { return x + y; }
B. async function fetchData() { const response = await fetch(“http://example.com/data”); const data = await response.json(); return data; }
C. var x = 5; x++;
Answer: B
- What is the output of console.log([1, 2, 3].reverse())?
A. [1, 2, 3]
B. [3, 2, 1]
C. TypeError
Answer: B
- Top 10 DevOps Trainers in the world - October 7, 2023
- What is Cookies and Why it is Used? - May 24, 2023
- TOP trends of transitions in TikTok - May 10, 2023