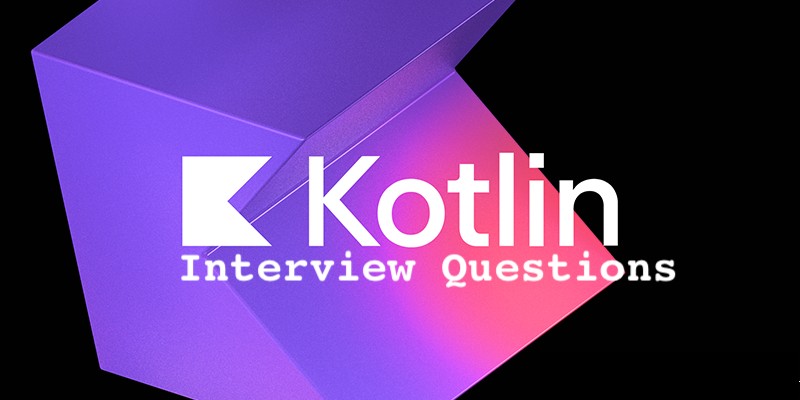
1. What is Kotlin?
a. An object-oriented programming language
b. A markup language
c. A database management system
d. A server-side framework
Answer: a. An object-oriented programming language
2. Which company developed Kotlin?
a. Google
b. JetBrains
c. Oracle
d. IBM
Answer: b. JetBrains
3. Which platform does Kotlin target?
a. iOS
b. Android
c. Windows
d. macOS
Answer: b. Android
4. What is null safety in Kotlin?
a. A feature that prevents NullPointerExceptions
b. A feature that allows only null values
c. A feature that enables dynamic typing
d. A feature that ensures thread safety
Answer: a. A feature that prevents NullPointerExceptions
5. What is the default visibility of a class declared in Kotlin?
a. Private
b. Public
c. Protected
d. Internal
Answer: d. Internal
6. What are the different types of constructors available in Kotlin?
a. Default constructor and secondary constructor
b. Primary constructor and inner constructor
c. Protected constructor and public constructor
d. Parent constructor and child constructor
Answer: a. Default constructor and secondary constructor
7. Which keyword is used to create a singleton class in Kotlin?
a. object
b. class
c. singleton
d. static
Answer: a. object
8. What is the use of a companion object in Kotlin?
a. To create a static method or variable
b. To create an instance of a class
c. To define a constructor
d. To declare a null object
Answer: a. To create a static method or variable
9. Which of the following is not a basic data type in Kotlin?
a. boolean
b. byte
c. char
d. float
Answer: d. float
10. Which keyword is used to create an abstract class in Kotlin?
a. abstract
b. class
c. abstracted
d. abc
Answer: a. abstract
11. What is the use of the operator keyword in Kotlin?
a. To overload an operator
b. To specify an algorithm
c. To define a function
d. To declare a variable
Answer: a. To overload an operator
12. What is the use of the lateinit keyword in Kotlin?
a. To declare a variable that will be initialized later
b. To declare a constant
c. To declare a nullable variable
d. To declare a final variable
Answer: a. To declare a variable that will be initialized later
13. Which keyword is used to declare a variable that cannot be reassigned?
a. val
b. var
c. const
d. final
Answer: a. val
14. What is the use of the safe call operator in Kotlin?
a. To avoid NullPointerExceptions
b. To access a variable
c. To define a function
d. To declare a class
Answer: a. To avoid NullPointerExceptions
15. Which function is used in Kotlin to convert a string to an integer?
a. toInt()
b. parseInt()
c. fromString()
d. convertInt()
Answer: a. toInt()
16. What is the use of the when expression in Kotlin?
a. To replace if-else statements
b. To declare a variable
c. To define a function
d. To access a class
Answer: a. To replace if-else statements
17. What is the use of the assert() function in Kotlin?
a. To check a condition and terminate the program if it is false
b. To print the value of a variable
c. To define a function
d. To declare a class
Answer: a. To check a condition and terminate the program if it is false
18. Which keyword is used to declare a function that doesn’t return any value?
a. void
b. Unit
c. fun
d. none
Answer: b. Unit
19. What is the use of the apply() function in Kotlin?
a. To initialize an object
b. To execute a block of code with a nullable variable
c. To inherit a class
d. To declare a constant
Answer: a. To initialize an object
20. What is the use of the with() function in Kotlin?
a. To execute a block of code with a nullable variable
b. To access a class
c. To declare a function
d. To define a constructor
Answer: a. To execute a block of code with a nullable variable
21. Which operator is used for safe navigation in Kotlin?
a. ?
b. !!
c. ?:
d. ?.
Answer: d. ?.
22. What is the use of the lazy() function in Kotlin?
a. To initialize a variable only when it is used for the first time
b. To declare a final variable
c. To declare a nullable variable
d. To define a function
Answer: a. To initialize a variable only when it is used for the first time
23. What is the use of the take() function in Kotlin?
a. To return a specified number of elements from a collection
b. To remove a specified number of elements from a collection
c. To sort a collection
d. To map a collection to a new collection
Answer: a. To return a specified number of elements from a collection
24. Which function is used to create a list in Kotlin?
a. arrayListOf()
b. listOf()
c. mutableListOf()
d. setOf()
Answer: b. listOf()
25. What is the use of the until keyword in Kotlin?
a. To specify a range of numbers
b. To access a class
c. To define a constructor
d. To declare a function
Answer: a. To specify a range of numbers
26. What is the use of the ?: operator in Kotlin?
a. To execute a block of code with a nullable variable
b. To access a class
c. To declare a function
d. To provide a default value for a nullable variable
Answer: d. To provide a default value for a nullable variable
27. What is the use of the ?.let() function in Kotlin?
a. To execute a block of code with a nullable variable
b. To provide a default value for a nullable variable
c. To map a collection to a new collection
d. To sort a collection
Answer: a. To execute a block of code with a nullable variable
28. Which function is used to create a set in Kotlin?
a. setOf()
b. mutableSetOf()
c. hashSetOf()
d. all of the above
Answer: d. all of the above
29. What is the use of the filter() function in Kotlin?
a. To return a new collection that contains all elements matching a given condition
b. To return a new collection that contains only the non-null elements of the original collection
c. To sort a collection
d. To map a collection to a new collection
Answer: a. To return a new collection that contains all elements matching a given condition
30. What is the use of the reduce() function in Kotlin?
a. To return a new collection that contains all elements matching a given condition
b. To return a new collection that contains only the non-null elements of the original collection
c. To combine the elements of a collection into a single value
d. To map a collection to a new collection
Answer: c. To combine the elements of a collection into a single value
31. What is the use of the fold() function in Kotlin?
a. To return a new collection that contains all elements matching a given condition
b. To return a new collection that contains only the non-null elements of the original collection
c. To combine the elements of a collection into a single value
d. To map a collection to a new collection
Answer: c. To combine the elements of a collection into a single value
32. What is the use of the distinct() function in Kotlin?
a. To return a new collection that contains only the unique elements of the original collection
b. To return a new collection that contains all elements matching a given condition
c. To combine the elements of a collection into a single value
d. To sort a collection
Answer: a. To return a new collection that contains only the unique elements of the original collection
33. What is the use of the groupBy() function in Kotlin?
a. To return a map of elements grouped by a given key
b. To return a new collection that contains all elements matching a given condition
c. To combine the elements of a collection into a single value
d. To sort a collection
Answer: a. To return a map of elements grouped by a given key
34. Which function is used to create a map in Kotlin?
a. mapOf()
b. hashMapOf()
c. mutableMapOf()
d. all of the above
Answer: d. all of the above
35. What is the use of the to() function in Kotlin?
a. To declare a variable
b. To define a function
c. To create a pair of values
d. To access a class
Answer: c. To create a pair of values
36. What is the use of the mutableListOf() function in Kotlin?
a. To create an immutable list
b. To create a mutable list
c. To create a set
d. To create a map
Answer: b. To create a mutable list
37. What is the use of the sorted() function in Kotlin?
a. To sort a collection in natural order
b. To sort a collection in reverse order
c. To shuffle a collection
d. To filter a collection
Answer: a. To sort a collection in natural order
38. What is the use of the reversed() function in Kotlin?
a. To sort a collection in natural order
b. To sort a collection in reverse order
c. To shuffle a collection
d. To filter a collection
Answer: b. To sort a collection in reverse order
39. What is the use of the shuffle() function in Kotlin?
a. To sort a collection in natural order
b. To sort a collection in reverse order
c. To shuffle a collection
d. To filter a collection
Answer: c. To shuffle a collection
40. What is the use of the takeWhile() function in Kotlin?
a. To return a new collection that contains all elements matching a given condition
b. To return a new collection that contains only the non-null elements of the original collection
c. To return a new collection that contains elements until a given condition is no longer met
d. To map a collection to a new collection
Answer: c. To return a new collection that contains elements until a given condition is no longer met
41. What is the use of the joinToString() function in Kotlin?
a. To convert a collection to a string
b. To filter a collection
c. To sort a collection
d. To map a collection to a new collection
Answer: a. To convert a collection to a string
42. What is the use of the let() function in Kotlin?
a. To execute a block of code with a nullable variable
b. To provide a default value for a nullable variable
c. To define a function
d. To access a class
Answer: a. To execute a block of code with a nullable variable
43. What is the use of the forEach() function in Kotlin?
a. To loop through a collection and perform an action on each element
b. To return a new collection that contains all elements matching a given condition
c. To combine the elements of a collection into a single value
d. To declare a variable
Answer: a. To loop through a collection and perform an action on each element
44. What is the use of the mapNotNull() function in Kotlin?
a. To return a new collection that contains only the non-null elements of the original collection
b. To return a new collection that contains all elements matching a given condition
c. To combine the elements of a collection into a single value
d. To map a collection to a new collection
Answer: a. To return a new collection that contains only the non-null elements of the original collection
45. What is the use of the zip() function in Kotlin?
a. To combine two collections into a single list of pairs
b. To filter a collection
c. To sort a collection
d. To map a collection to a new collection
Answer: a. To combine two collections into a single list of pairs
46. What is the use of the flatMap() function in Kotlin?
a. To map a collection to a new collection
b. To combine nested collections into a single collection
c. To sort a collection
d. To filter a collection
Answer: b. To combine nested collections into a single collection
47. What is the use of the distinctBy() function in Kotlin?
a. To return a new collection that contains all elements matching a given condition
b. To return a new collection that contains only the unique elements of the original collection based on a key selector
c. To combine the elements of a collection into a single value
d. To sort a collection
Answer: b. To return a new collection that contains only the unique elements of the original collection based on a key selector
48. What is the use of the sortedBy() function in Kotlin?
a. To sort a collection in natural order based on a key selector
b. To sort a collection in reverse order based on a key selector
c. To shuffle a collection based on a key selector
d. To filter a collection based on a key selector
Answer: a. To sort a collection in natural order based on a key selector
49. What is the use of the associateBy() function in Kotlin?
a. To filter a collection based on a key selector
b. To sort a collection based on a key selector
c. To map a collection to a new collection with keys based on a key selector
d. To combine the elements of a collection into a single value based on a key selector
Answer: c. To map a collection to a new collection with keys based on a key selector
50. What is the use of the mapKeys() function in Kotlin?
a. To filter a collection based on a key selector
b. To sort a collection based on a key selector
c. To map a collection to a new collection with keys based on a key selector
d. To combine the elements of a collection into a single value based on a key selector
Answer: c. To map a collection to a new collection with keys based on a key selector
- Top 10 DevOps Trainers in the world - October 7, 2023
- What is Cookies and Why it is Used? - May 24, 2023
- TOP trends of transitions in TikTok - May 10, 2023