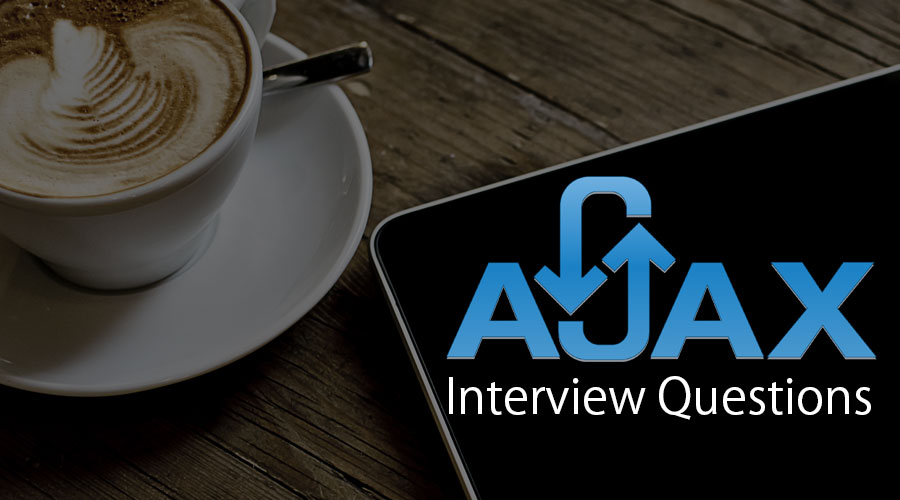
1. What is AJAX?
A. A programming language
B. A web development technique
C. A database management system
Answer: B
2. What is the full form of AJAX?
A. Asynchronous JavaScript and XML
B. Asynchronous JavaScript and XHTML
C. Asynchronous JavaScript and JSON
Answer: A
3. What is the main advantage of using AJAX?
A. Improved page load time
B. Improved database performance
C. Improved server response time
Answer: A
4. Which HTTP request method is used in AJAX?
A. GET
B. POST
C. Both A and B
Answer: C
5. Which object is used to create an AJAX request?
A. XMLHttpRequest
B. JSONRequest
C. AjaxRequest
Answer: A
6. What is the role of JSON in AJAX?
A. To store data
B. To retrieve data
C. To exchange data
Answer: C
7. Which function is used to send an AJAX request?
A. xhr.send()
B. xhr.open()
C. xhr.onload()
Answer: A
8. Which function is used to handle AJAX response?
A. xhr.onload()
B. xhr.open()
C. xhr.send()
Answer: A
9. Which HTML tag is used to create an AJAX application?
A.
B.
C.
Answer: C
10. Which file is used to handle AJAX requests in PHP?
A. ajax.php
B. ajax_handler.php
C. any PHP file can handle AJAX requests
Answer: C
11. Which function is used to encode a JavaScript object into JSON format?
A. JSON.format()
B. JSON.encode()
C. JSON.stringify()
Answer: C
12. Which function is used to decode a JSON string into a JavaScript object?
A. JSON.parse()
B. JSON.decode()
C. JSON.object()
Answer: A
13. Which method is used to append data to a form before submitting it using AJAX?
A. xhr.append()
B. xhr.send()
C. FormData.append()
Answer: C
14. How can you handle errors in AJAX requests?
A. Using try-catch block
B. Using xhr.onerror()
C. Both A and B
Answer: C
15. Which web development framework has built-in support for AJAX?
A. React
B. AngularJS
C. jQuery
Answer: C
16. What is the purpose of the XMLHttpRequest.readyState property in AJAX?
A. To indicate the status of the AJAX request
B. To handle AJAX response
C. To create an AJAX request
Answer: A
17. Which HTTP status code indicates a successful AJAX request?
A. 200
B. 400
C. 500
Answer: A
18. Which method is used to cancel an AJAX request?
A. xhr.cancel()
B. xhr.abort()
C. xhr.stop()
Answer: B
19. Which method is used to set a timeout for an AJAX request?
A. xhr.timeout()
B. xhr.setOnTimeout()
C. xhr.ontimeout()
Answer: C
20. Which method is used to disable caching for an AJAX request?
A. xhr.disableCache()
B. xhr.setRequestHeader()
C. xhr.cache(false)
Answer: B
21. What is the syntax for creating an AJAX request using jQuery?
A. $.ajax(url, options)
B. $.getJSON(url, data)
C. $.post(url, data)
Answer: A
22. Which method is used to set the data type of an AJAX response in jQuery?
A. dataType()
B. responseType()
C. contentType()
Answer: A
23. Which property is used to access the response data in an AJAX request using jQuery?
A. data
B. responseText
C. responseJSON
Answer: A
24. Which method is used to serialize a form data in jQuery?
A. serializeForm()
B. serializeObject()
C. serialize()
Answer: C
25. Which method is used to submit a form using AJAX in jQuery?
A. ajax()
B. submit()
C. post()
Answer: A
26. What is the syntax for creating an AJAX request using React?
A. fetch(url, options)
B. axios(url, options)
C. XMLHttpRequest(url, options)
Answer: B
27. Which method is used to handle errors in an AJAX request using React?
A. onError()
B. onFailure()
C. catch()
Answer: C
28. What is the purpose of the withCredentials property in AJAX requests?
A. To enable cookies in cross-domain requests
B. To set the credentials for authentication
C. To enable HTTPS requests
Answer: A
29. Which method is used to enable cross-domain requests in AJAX?
A. xhr.enableCORS()
B. xhr.crossDomain()
C. xhr.setRequestHeader()
Answer: C
30. What is the syntax for creating an AJAX request using AngularJS?
A. $http.get(url, options)
B. $ajax(url, options)
C. $xhr(url, options)
Answer: A
31. Which method is used to handle an error in an AJAX request using AngularJS?
A. error()
B. catch()
C. failure()
Answer: A
32. What is the purpose of the transformRequest function in AngularJS AJAX requests?
A. To transform the request data before sending
B. To transform the response data after receiving
C. To transform the HTTP headers
Answer: A
33. What is the purpose of the transformResponse function in AngularJS AJAX requests?
A. To transform the request data before sending
B. To transform the response data after receiving
C. To transform the HTTP headers
Answer: B
34. Which method is used to cancel an AJAX request in AngularJS?
A. $cancel()
B. $abort()
C. $http.abort()
Answer: A
35. What is the syntax for creating an AJAX request using Vue.js?
A. axios(url, options)
B. $xhr(url, options)
C. fetch(url, options)
Answer: A
36. Which method is used to handle an error in an AJAX request using Vue.js?
A. onError()
B. onFailure()
C. catch()
Answer: C
37. What is the purpose of the beforeRequest hook in Vue.js AJAX requests?
A. To modify the request before sending
B. To modify the response after receiving
C. To modify the HTTP headers
Answer: A
38. What is the purpose of the afterResponse hook in Vue.js AJAX requests?
A. To modify the request before sending
B. To modify the response after receiving
C. To modify the HTTP headers
Answer: B
39. Which method is used to cancel an AJAX request in Vue.js?
A. $cancel()
B. $abort()
C. axios.cancel()
Answer: A
40. Which HTTP headers are used to enable caching in AJAX requests?
A. Cache-Control and Expires
B. Last-Modified and ETag
C. Both A and B
Answer: C
41. What is the purpose of ETag header in HTTP responses?
A. To store cached data
B. To enable caching for dynamic content
C. To validate cached data
Answer: C
42. What is the difference between synchronous and asynchronous AJAX requests?
A. Synchronous requests are faster than asynchronous requests
B. Asynchronous requests do not block the UI thread
C. Synchronous requests do not require a callback function
Answer: B
43. What is the purpose of CORS in AJAX requests?
A. To enable cross-domain requests
B. To disable cross-domain requests
C. To validate cross-domain requests
Answer: A
44. Which web security vulnerability is associated with AJAX?
A. Cross-site scripting (XSS)
B. Cross-site request forgery (CSRF)
C. SQL injection
Answer: B
45. What is the purpose of using a nonce in AJAX requests?
A. To prevent CSRF attacks
B. To enable caching
C. To improve server performance
Answer: A
46. Which method is used to encrypt AJAX requests over HTTPS?
A. xhr.encrypt()
B. xhr.setSSL()
C. xhr.setRequestHeader()
Answer: C
47. What is the purpose of the HTTP referer header in AJAX requests?
A. To trace the origin of the request
B. To identify the user agent
C. To set the caching policy
Answer: A
48. What is the purpose of the HTTP user agent header in AJAX requests?
A. To trace the origin of the request
B. To identify the user agent
C. To set the caching policy
Answer: B
49. Which method is used to detect the presence of an AJAX request in JavaScript?
A. xhr.detect()
B. xhr.status()
C. xhr.readyState()
Answer: C
50. Which web development standard is used for AJAX requests?
A. HTTP
B. XML
C. JSON