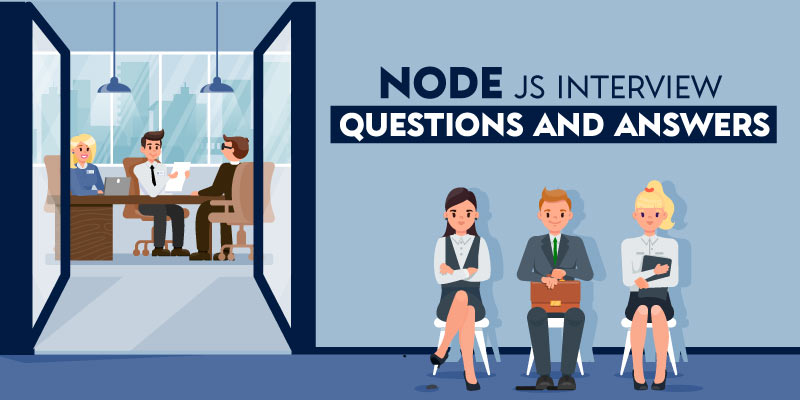
1. What is Node.js?
a. A programming language
b. A web server
c. A runtime environment for JavaScript
d. A database management system
Answer: c
2. Which event loop does Node.js use?
a. Non-blocking
b. Blocking
c. Sequential
d. Parallel
Answer: a
3. What is the purpose of the Node.js modules?
a. To create reusable code
b. To import JavaScript libraries
c. To add new features to Node.js
d. To improve Node.js performance
Answer: a
4. What is callback hell?
a. When a function calls itself recursively
b. When too many callbacks are nested within one another
c. When a callback function returns an error
d. When a callback function takes too long to execute
Answer: b
5. What is the purpose of the Node.js package.json file?
a. To store metadata about the project
b. To store the project’s source code
c. To store the project’s dependencies
d. To store the project’s configuration settings
Answer: c
6. What is NPM?
a. Node Package Manager
b. Node Programming Module
c. Node Project Manager
d. Node Package Module
Answer: a
7. How can you install a Node.js module using NPM?
a. npm install module-name
b. node install module-name
c. module-name install npm
d. install npm module-name
Answer: a
8. What is a buffer in Node.js?
a. A temporary storage area for data
b. A data type used to represent binary data
c. A function used to read and write files
d. An object used to create streams
Answer: b
9. What is middleware in Node.js?
a. A function that is executed before processing a request
b. A function that is executed after processing a request
c. A function that is executed between two other functions
d. A function that is executed when an error occurs
Answer: c
10. What is the purpose of the Node.js http module?
a. To handle HTTP requests and responses
b. To create and manage web servers
c. To send emails
d. To connect to a database
Answer: a
11. Which HTTP method is used to update a resource?
a. GET
b. POST
c. PUT
d. DELETE
Answer: c
12. What is the difference between res.send() and res.end() methods in Node.js?
a. res.send() sends JSON data, while res.end() sends plain text
b. res.send() sends plain text, while res.end() sends JSON data
c. res.send() terminates the response, while res.end() does not
d. res.send() and res.end() are interchangeable
Answer: a
13. What is an EventEmitter in Node.js?
a. A function that listens for events
b. An object that emits events
c. A method that triggers an event
d. A module that handles events
Answer: b
14. What is the purpose of the fs module in Node.js?
a. To handle file system operations
b. To create and manage web servers
c. To send emails
d. To connect to a database
Answer: a
15. What is a route in Node.js?
a. A path to a file
b. A URL endpoint
c. A function that handles a specific HTTP request
d. A function that handles a specific HTTP response
Answer: b
16. What is Express.js?
a. A library for building web applications in Node.js
b. A Node.js module for handling HTTP requests and responses
c. An object-oriented programming language
d. A database management system
Answer: a
17. What is Mongoose?
a. A Node.js module for working with MongoDB
b. A Node.js module for working with MySQL
c. A web server framework for Node.js
d. A front-end framework for Node.js
Answer: a
18. What is the difference between MongoDB and MySQL?
a. MongoDB is a document-oriented database, while MySQL is a relational database
b. MongoDB is open source, while MySQL is not
c. MongoDB is faster than MySQL
d. MySQL is more scalable than MongoDB
Answer: a
19. What is the purpose of the cluster module in Node.js?
a. To create multiple child processes of a Node.js application
b. To create a cluster of web servers
c. To manage user sessions
d. To handle database connections
Answer: a
20. What is the purpose of the bcrypt module in Node.js?
a. To create and manage web servers
b. To encrypt and decrypt data
c. To handle file system operations
d. To connect to a database
Answer: b
21. What is the purpose of the Node.js net module?
a. To handle HTTP requests and responses
b. To handle TCP connections
c. To send emails
d. To connect to a database
Answer: b
22. What is the purpose of the forever module in Node.js?
a. To create and manage web servers
b. To continuously run a Node.js application
c. To handle file system operations
d. To connect to a database
Answer: b
23. What is the purpose of the Node.js child_process module?
a. To handle file system operations
b. To create and manage child processes
c. To handle HTTP requests and responses
d. To manage user sessions
Answer: b
24. What is a stream in Node.js?
a. A data type used to represent binary data
b. A buffer that is read or written to sequentially
c. A function that processes data asynchronously
d. An object that is used to create streams
Answer: b
25. What is the purpose of the Node.js util module?
a. To handle file system operations
b. To create and manage web servers
c. To provide utility functions
d. To connect to a database
Answer: c
26. What is a Readable stream in Node.js?
a. A stream that can only be read from
b. A stream that can only be written to
c. A stream that can be both read from and written to
d. A stream that is used to read and write files
Answer: a
27. What is a Writable stream in Node.js?
a. A stream that can only be read from
b. A stream that can only be written to
c. A stream that can be both read from and written to
d. A stream that is used to read and write files
Answer: b
28. What is the purpose of the os module in Node.js?
a. To handle file system operations
b. To create and manage web servers
c. To interact with the operating system
d. To connect to a database
Answer: c
29. What is the purpose of the http-proxy module in Node.js?
a. To handle HTTP requests and responses
b. To create and manage web servers
c. To proxy HTTP requests to other servers
d. To connect to a database
Answer: c
30. What is the purpose of the Node.js crypto module?
a. To handle file system operations
b. To create and manage web servers
c. To encrypt and decrypt data
d. To connect to a database
Answer: c
31. What is the purpose of the Node.js path module?
a. To provide utility functions for working with file paths
b. To create and manage web servers
c. To handle HTTP requests and responses
d. To connect to a database
Answer: a
32. What is the purpose of the Node.js url module?
a. To create and manage web servers
b. To handle HTTP requests and responses
c. To parse and format URLs
d. To connect to a database
Answer: c
33. What is the purpose of the Node.js zlib module?
a. To handle file system operations
b. To create and manage web servers
c. To compress and decompress data
d. To connect to a database
Answer: c
34. What is the purpose of the Node.js cluster module?
a. To create and manage child processes of a Node.js application
b. To create a cluster of web servers
c. To handle database connections
d. To handle user authentication and authorization
Answer: a
35. What is a Promise in Node.js?
a. A function that is executed before processing a request
b. An object that represents the eventual completion of an asynchronous operation
c. A method that triggers an event
d. A method that handles rejected Promises
Answer: b
36. What is the purpose of the Node.js assert module?
a. To handle HTTP requests and responses
b. To create and manage web servers
c. To write unit tests for Node.js applications
d. To connect to a database
Answer: c
37. What is the purpose of the Node.js debug module?
a. To create and manage web servers
b. To handle database connections
c. To improve the debugging capabilities of Node.js
d. To handle file system operations
Answer: c
38. What is the purpose of the Node.js dgram module?
a. To handle HTTP requests and responses
b. To handle datagram sockets
c. To create and manage web servers
d. To connect to a database
Answer: b
39. What is the purpose of the Node.js punycode module?
a. To encode and decode domain names
b. To create and manage web servers
c. To handle file system operations
d. To connect to a database
Answer: a
40. What is the purpose of the Node.js q module?
a. To create and manage web servers
b. To provide a simple and powerful promise-based API
c. To handle file system operations
d. To connect to a database
Answer: b
41. What is the purpose of the Node.js request module?
a. To handle HTTP requests and responses
b. To create and manage web servers
c. To handle file system operations
d. To connect to a database
Answer: a
42. What is the purpose of the Node.js sinon module?
a. To create and manage web servers
b. To improve the testing capabilities of Node.js
c. To handle file system operations
d. To connect to a database
Answer: b
43. What is a duplex stream in Node.js?
a. A stream that can only be read from
b. A stream that can only be written to
c. A stream that can be both read from and written to
d. A stream that is used to read and write files
Answer: c
44. What is the purpose of the Node.js tty module?
a. To handle HTTP requests and responses
b. To create and manage web servers
c. To interact with the terminal
d. To connect to a database
Answer: c
45. What is the purpose of the Node.js zlib-sync module?
a. To handle file system operations
b. To compress and decompress data synchronously
c. To handle HTTP requests and responses
d. To connect to a database
Answer: b
46. What is the purpose of the Node.js async module?
a. To provide a simple and powerful promise-based API
b. To handle file system operations
c. To create and manage web servers
d. To provide utility functions for working with asynchronous code
Answer: d
47. What is the purpose of the Node.js strong-agent module?
a. To create and manage web servers
b. To improve the performance of Node.js applications
c. To handle file system operations
d. To connect to a database
Answer: b
48. What is the purpose of the Node.js chokidar module?
a. To watch files and directories for changes
b. To create and manage web servers
c. To handle HTTP requests and responses
d. To connect to a database
Answer: a
49. What is the purpose of the Node.js fs-extra module?
a. To handle file system operations
b. To create and manage web servers
c. To handle HTTP requests and responses
d. To connect to a database
Answer: a
50. What is the purpose of the Node.js clusterize module?
a. To create and manage child processes of a Node.js application
b. To improve the scalability of a Node.js application
c. To handle database connections
d. To handle user authentication and authorization
Answer: b
- Top 10 DevOps Trainers in the world - October 7, 2023
- What is Cookies and Why it is Used? - May 24, 2023
- TOP trends of transitions in TikTok - May 10, 2023