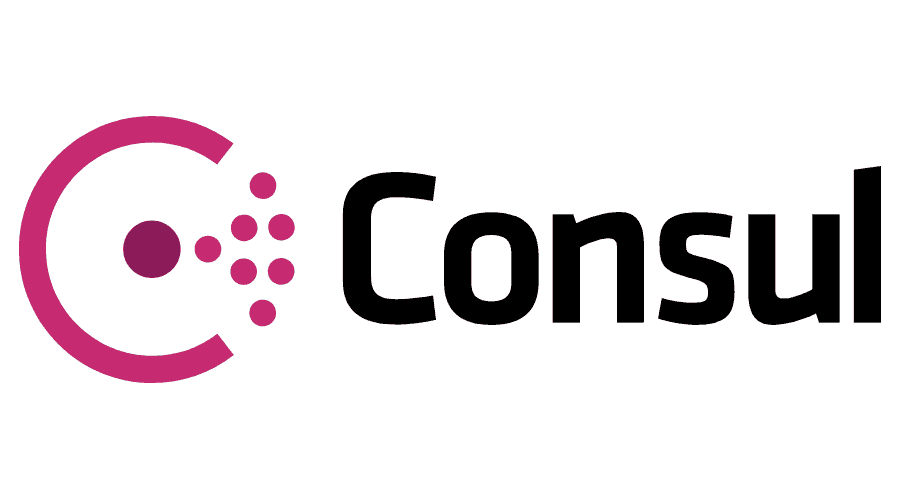
1. What is the difference between getch() and getche() functions?
A) getch() prints the character on the screen and getche() doesn’t
B) getche() waits for a key input and getch() doesn’t
C) getch() doesn’t wait for a key input and getche() does
D) None of the above
Answer: C)
2. What is a pointer?
A) A variable that stores the address of another variable
B) A variable that stores the value of another variable
C) A variable that stores the address of a function
D) None of the above
Answer: A)
3. What is the difference between malloc() and calloc() functions?
A) malloc() doesn’t initialize the memory and calloc() does
B) calloc() doesn’t initialize the memory and malloc() does
C) Both malloc() and calloc() initialize the memory
D) None of the above
Answer: A)
4. What is recursion?
A) A function calling itself
B) A function calling another function
C) A variable calling another variable
D) None of the above
Answer: A)
5. What is a structure in C?
A) A data type that contains multiple variables
B) A function that contains multiple variables
C) A loop that contains multiple variables
D) None of the above
Answer: A)
6. What is the difference between local and global variables?
A) Local variables are initialized in the main function and global variables are initialized outside the main function
B) Global variables are initialized in the main function and local variables are initialized outside the main function
C) Local variables are only accessible within the function in which they are declared, while global variables can be accessed anywhere in the program
D) None of the above
Answer: C)
7. What is the purpose of the ‘typedef’ keyword?
A) To define new data types
B) To declare a variable
C) To declare a function
D) None of the above
Answer: A)
8. Who is the founder of the C programming language?
A) Tim Berners-Lee
B) Dennis Ritchie
C) Bill Gates
D) None of the above
Answer: B)
9. What is the maximum value of an integer in C?
A) 2^15
B) 2^31
C) 2^63
D) None of the above
Answer: B)
10. What is the function of the ‘volatile’ keyword in C?
A) To declare a variable
B) To inform the compiler that the value of the variable may change at any time
C) To inform the programmer that the value of the variable cannot change
D) None of the above
Answer: B)
11. What is a static variable in C?
A) A variable that is declared inside a function
B) A variable that retains its value through function calls
C) A variable that can be accessed outside the function in which it is declared
D) None of the above
Answer: B)
12. What is a union in C?
A) A data type that allows multiple values to be stored in the same memory location
B) A data type that allows multiple variables to be stored in the same memory location
C) A data type that allows multiple functions to be stored in the same memory location
D) None of the above
Answer: A)
13. What is the difference between ‘++i’ and ‘i++’?
A) ‘++i’ increments the value of i by 1 before the expression is evaluated and ‘i++’ increments the value of i by 1 after the expression is evaluated
B) ‘++i’ increments the value of i by 2 before the expression is evaluated and ‘i++’ increments the value of i by 2 after the expression is evaluated
C) ‘++i’ and ‘i++’ are the same thing
D) None of the above
Answer: A)
14. What is an enumeration in C?
A) A data type that allows a variable to hold a range of values
B) A data type that allows a variable to hold a boolean value
C) A data type that allows a variable to hold an integer value
D) None of the above
Answer: C)
15. What is the difference between a macro and a function in C?
A) A macro is a block of code that is replaced by its value when it is called, while a function is a separate entity that is called
B) A function is a block of code that is replaced by its value when it is called, while a macro is a separate entity that is called
C) A macro is defined using the ‘#define’ directive, while a function is defined using the ‘def’ keyword
D) None of the above
Answer: A)
16. What is a file pointer in C?
A) A pointer that points to a function
B) A pointer that points to a variable
C) A pointer that points to a structure
D) A pointer that points to a file
Answer: D)
17. What is the difference between ‘fgets()’ and ‘gets()’?
A) ‘fgets()’ reads a line of text from a file and ‘gets()’ reads a line of text from the keyboard
B) ‘fgets()’ reads a line of text from the keyboard and ‘gets()’ reads a line of text from a file
C) ‘fgets()’ is safer than ‘gets()’ because it limits the number of characters that can be read, while ‘gets()’ does not
D) None of the above
Answer: C)
18. What is the difference between a signed and an unsigned integer?
A) A signed integer can hold both negative and positive values, while an unsigned integer can only hold positive values
B) A signed integer can only hold negative values, while an unsigned integer can hold both negative and positive values
C) Both signed and unsigned integers can hold both negative and positive values
D) None of the above
Answer: A)
19. What is the use of the ‘const’ keyword in C?
A) To declare a variable as mutable
B) To declare a variable as immutable
C) To declare a variable as volatile
D) None of the above
Answer: B)
20. What is the difference between ‘break’ and ‘continue’ statements?
A) ‘break’ stops the execution of the loop, while ‘continue’ skips to the next iteration of the loop
B) ‘continue’ stops the execution of the loop, while ‘break’ skips to the next iteration of the loop
C) ‘break’ and ‘continue’ are the same thing
D) None of the above
Answer: A)
21. What is the difference between ‘char’ and ‘int’ data types?
A) ‘char’ is used to store characters, while ‘int’ is used to store integers
B) ‘char’ is 1 byte in size, while ‘int’ is 2 bytes in size
C) ‘char’ can hold a maximum value of 127, while ‘int’ can hold a maximum value of 32,767
D) None of the above
Answer: A)
22. What is a function pointer in C?
A) A pointer that points to a function
B) A pointer that points to a variable
C) A pointer that points to a structure
D) None of the above
Answer: A)
23. What is the difference between ‘%d’ and ‘%f’ in printf() function?
A) ‘%d’ is used to print integers, while ‘%f’ is used to print characters
B) ‘%d’ is used to print integers, while ‘%f’ is used to print floats
C) Both ‘%d’ and ‘%f’ are used to print integers
D) None of the above
Answer: B)
24. What is the difference between ‘strcpy()’ and ‘strncpy()’ functions?
A) ‘strcpy()’ copies the entire string, while ‘strncpy()’ copies the string up to a specified length
B) ‘strncpy()’ copies the entire string, while ‘strcpy()’ copies the string up to a specified length
C) ‘strcpy()’ and ‘strncpy()’ are the same thing
D) None of the above
Answer: A)
25. What is the difference between ‘&’ and ‘*’ in C?
A) ‘&’ is used to get the address of a variable, while ‘’ is used to dereference a pointer B) ‘&’ is used to dereference a pointer, while ‘’ is used to get the address of a variable
C) ‘&’ and ‘*’ are the same thing
D) None of the above
Answer: A)
26. What is the purpose of the ‘goto’ statement in C?
A) To jump to a specific label within the code
B) To end the current loop and move on to the next iteration
C) To print a message on the screen
D) None of the above
Answer: A)
27. What is a double data type in C?
A) A data type that stores decimal numbers with single precision
B) A data type that stores decimal numbers with double precision
C) A data type that stores integers with double precision
D) None of the above
Answer: B)
28. What is a null pointer in C?
A) A pointer that points to a valid memory location
B) A pointer that points to an invalid memory location
C) A pointer that points to a null value
D) None of the above
Answer: C)
29. What is a multi-dimensional array in C?
A) An array that can hold multiple values of different data types
B) An array that can hold multiple values of the same data type
C) An array that has more than one dimension
D) None of the above
Answer: C)
30. What is the difference between ‘void main()’ and ‘int main()’?
A) ‘void main()’ doesn’t return a value, while ‘int main()’ returns an integer value
B) ‘int main()’ doesn’t return a value, while ‘void main()’ returns a void value
C) ‘void main()’ and ‘int main()’ are the same thing
D) None of the above
Answer: A)
31. What is the difference between ‘scanf()’ and ‘fgets()’ functions?
A) ‘scanf()’ reads a line of text from the keyboard and ‘fgets()’ reads a line of text from a file
B) ‘fgets()’ reads a line of text from the keyboard and ‘scanf()’ reads a line of text from a file
C) ‘scanf()’ can read values of different data types, while ‘fgets()’ can only read strings
D) None of the above
Answer: C)
32. What is the difference between structure and union in C programming?
A) A structure can hold variables of different data types, while a union can hold variables of the same data type
B) A structure can hold variables of the same data type, while a union can hold variables of different data types
C) Structure and union are the same thing
D) None of the above
Answer: A)
33. What is a heap in C?
A) A memory that is dynamically allocated and de-allocated during the program execution
B) A memory that is statically allocated and de-allocated during the program execution
C) A memory that is allocated and de-allocated by the operating system
D) None of the above
Answer: A)
34. What is a bit-field in C?
A) A data type that can hold bits
B) A variable that is used to access bits of a larger data type
C) A data type that allows multiple variables to be stored in the same memory location
D) None of the above
Answer: B)
35. What is a pointer to a function in C?
A) A pointer that points to a variable
B) A pointer that points to a structure
C) A pointer that points to a specific function
D) None of the above
Answer: C)
36. What is the difference between a stack and a heap?
A) A stack is a fixed size memory region, while a heap is a dynamically allocated memory region
B) A stack is used for static memory allocation, while a heap is used for dynamic memory allocation
C) A stack follows a LIFO (Last In First Out) structure, while a heap does not follow any specific structure
D) None of the above
Answer: A)
37. What is the difference between ‘->’ and ‘.’ operators in C?
A) ‘->’ is used to access a member of a structure or union through a pointer, while ‘.’ is used to access a member of a structure or union directly
B) ‘.’ is used to access a member of a structure or union through a pointer, while ‘->’ is used to access a member of a structure or union directly
C) ‘->’ and ‘.’ are the same thing
D) None of the above
Answer: A)
38. What is a typedef in C?
A) A keyword that creates an alias for a data type
B) A keyword that creates an alias for a variable
C) A keyword that creates an alias for a function
D) None of the above
Answer: A)
39. What is the difference between ‘const char *’ and ‘char const *’?
A) ‘const char *’ is a pointer to a constant character, while ‘char const *’ is a constant pointer to a character
B) ‘char const *’ is a pointer to a constant character, while ‘const char *’ is a constant pointer to a character
C) ‘const char *’ and ‘char const *’ are the same thing
D) None of the above
Answer: B)
40. What is the difference between ‘rand()’ and ‘srand()’ functions?
A) ‘rand()’ generates a random number, while ‘srand()’ sets the seed value for the random number generator
B) ‘srand()’ generates a random number, while ‘rand()’ sets the seed value for the random number generator
C) ‘rand()’ and ‘srand()’ are the same thing
D) None of the above
Answer: A)
41. What is the difference between ‘++p’ and ‘p++’?
A) ‘++p’ increments the value of the pointer by 1, while ‘p++’ increments the value of the pointer by the size of the data type
B) ‘p++’ increments the value of the pointer by 1, while ‘++p’ increments the value of the pointer by the size of the data type
C) ‘++p’ and ‘p++’ are the same thing
D) None of the above
Answer: A)
42. What is the difference between ‘calloc()’ and ‘realloc()’ functions?
A) ‘calloc()’ is used to allocate memory and initialize it to 0, while ‘realloc()’ is used to reallocate memory
B) ‘realloc()’ is used to allocate memory and initialize it to 0, while ‘calloc()’ is used to reallocate memory
C) Both ‘calloc()’ and ‘realloc()’ are used to allocate memory
D) None of the above
Answer: A)
43. What is a linked list in C?
A) A data structure that consists of a sequence of nodes, where each node contains a data value and a pointer to the next node
B) A data structure that consists of a two-dimensional array
C) A data structure that consists of a single variable
D) None of the above
Answer: A)
44. What is the difference between structure and array in C?
A) A structure can hold variables of different data types, while an array can hold variables of the same data type
B) A structure can hold variables of the same data type, while an array can hold variables of different data types
C) Structure and array are the same thing
D) None of the above
Answer: A)
45. What is the difference between ‘const’ and ‘volatile’ keywords in C?
A) ‘const’ keyword is used to declare a variable as immutable, while ‘volatile’ keyword is used to declare a variable as potentially changeable
B) ‘volatile’ keyword is used to declare a variable as immutable, while ‘const’ keyword is used to declare a variable as potentially changeable
C) ‘const’ and ‘volatile’ keywords are the same thing
D) None of the above
Answer: A)
46. What is an enum in C?
A) A data type that allows a variable to hold a range of values
B) A data type that allows a variable to hold a boolean value
C) A data type that allows a variable to hold an integer value
D) None of the above
Answer: C)
47. What is the difference between ‘#include ’ and ‘#include “file”‘ ?
A) ‘#include ’ searches for the file in the standard include path, while ‘#include “file”‘ searches for the file in the current directory
B) ‘#include “file”‘ searches for the file in the standard include path, while ‘#include ’ searches for the file in the current directory
C) ‘#include ’ and ‘#include “file”‘ are the same thing
D) None of the above
Answer: A)
48. What is the difference between ‘strcpy()’ and ‘sprintf()’ functions?
A) ‘strcpy()’ is used to copy strings, while ‘sprintf()’ is used to format strings
B) ‘sprintf()’ is used to copy strings, while ‘strcpy()’ is used to format strings
C) ‘strcpy()’ and ‘sprintf()’ are the same thing
D) None of the above
Answer: A)
49. What is the difference between ‘tolower()’ and ‘toupper()’ functions?
A) ‘tolower()’ converts a character to lowercase, while ‘toupper()’ converts a character to uppercase
B) ‘toupper()’ converts a character to lowercase, while ‘tolower()’ converts a character to uppercase
C) ‘tolower()’ and ‘toupper()’ are the same thing
D) None of the above
Answer: A)
50. What is a header file in C?
A) A file that contains function prototypes and macro definitions
B) A file that contains executable code
C) A file that contains variable declarations
D) None of the above