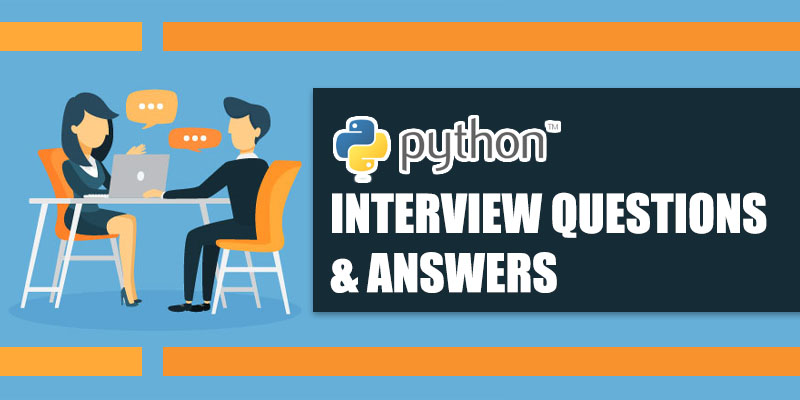
1) Which of the following is not a data type in Python?
a) Numbers
b) Strings
c) Lists
d) Characters
Answer: d) Characters
2) Which of the following is a built-in function in Python for sorting?
a) sort()
b) cmp()
c) sorted()
d) filter()
Answer: c) sorted()
3) What is the output of 2 ** 3?
a) 5
b) 6
c) 8
d) 9
Answer: c) 8
4) What does the “/” operator do in Python?
a) Divide two numbers and return the floating-point result
b) Divide two numbers and return the integer result
c) Multiply two numbers
d) None of the above
Answer: a) Divide two numbers and return the floating-point result
5) Which of the following is a loop in Python?
a) for loop
b) while loop
c) do-while loop
d) All of the above
Answer: d) All of the above
6) What is the output of the following code?
nums = [1, 2, 3, 4]
nums.append(5)
print(len(nums))
a) 4
b) 5
c) 6
d) None of the above
Answer: b) 5
7) Which of the following is used for multi-line comments in Python?
a) //
b) #
c) /*
d) “””
Answer: d) “””
8) What is the output of the following code?
num = 5
print(num*2)
a) 10
b) 5
c) 25
d) None of the above
Answer: a) 10
9) What is the output of the following code?
age = “18”
print(“My age is ” + age)
a) My age is 18
b) My age is “18”
c) 18
d) None of the above
Answer: b) My age is “18”
10) Which of the following is not a keyword in Python?
a) break
b) pass
c) continue
d) iterate
Answer: d) iterate
11) What is the output of the following code?
a = [1, 2, 3]
b = a
b[0] = 4
print(a)
a) [4, 2, 3]
b) [1, 2, 3]
c) [4, 4, 3]
d) None of the above
Answer: a) [4, 2, 3]
12) Which of the following is a data structure in Python?
a) Tuple
b) Vector
c) Map
d) Stack
Answer: a) Tuple
13) What is the output of the following code?
print(“Hello” + 5)
a) Hello5
b) 5Hello
c) Error
d) None of the above
Answer: c) Error
14) What is the output of the following code?
def square(x):
return x*x
print(square(5))
a) 10
b) 25
c) 50
d) None of the above
Answer: b) 25
15) Which of the following is used to find the length of a list in Python?
a) length()
b) count()
c) size()
d) len()
Answer: d) len()
16) What is the output of the following code?
a = “Hello”
print(a[1:3])
a) He
b) el
c) llo
d) None of the above
Answer: b) el
17) What is the output of the following code?
a = 4
if a > 5:
print(“a is greater than 5”)
else:
print(“a is less than or equal to 5”)
a) A is greater than 5
b) A is less than or equal to 5
c) Error
d) None of the above
Answer: b) A is less than or equal to 5
18) Which of the following is used for conditional statements in Python?
a) if-else
b) switch-case
c) for loop
d) while loop
Answer: a) if-else
19) What is the output of the following code?
a = [1, 2, 3]
b = [4, 5, 6]
print(a + b)
a) [1, 2, 3, 4, 5, 6]
b) [5, 7, 9]
c) Error
d) None of the above
Answer: a) [1, 2, 3, 4, 5, 6]
20) What is the output of the following code?
a = “Hello”
print(a.upper())
a) hello
b) HELLO
c) hELLO
d) None of the above
Answer: b) HELLO
21) Which of the following is used for defining a function in Python?
a) def
b) func
c) define
d) function
Answer: a) def
22) What is the output of the following code?
a = [1, 2, 3]
a.remove(2)
print(a)
a) [1, 3]
b) [1, 2, 3]
c) [2, 3]
d) None of the above
Answer: a) [1, 3]
23) Which of the following is used for importing modules in Python?
a) include
b) make
c) import
d) define
Answer: c) import
24) What is the output of the following code?
a = [1, 2, 3]
a.pop()
print(a)
a) [1, 2]
b) [1, 2, 3]
c) [2, 3]
d) None of the above
Answer: a) [1, 2]
25) Which of the following is used for creating a dictionary in Python?
a) {}
b) []
c) ()
d) //
Answer: a) {}
26) What is the output of the following code?
a = [1, 2, 3]
b = a.copy()
b[0] = 4
print(a)
a) [1, 2, 3]
b) [4, 2, 3]
c) [1, 4, 3]
d) None of the above
Answer: a) [1, 2, 3]
27) What is the output of the following code?
a = (1, 2, 3)
a[1] = 4
print(a)
a) (1, 2, 3)
b) (1, 4, 3)
c) Error
d) None of the above
Answer: c) Error
28) What is the output of the following code?
a = “Hello”
b = a.replace(“l”, “L”)
print(b)
a) Hello
b) HelLo
c) HELO
d) None of the above
Answer: b) HelLo
29) Which of the following is used for adding elements to a list in Python?
a) add()
b) insert()
c) put()
d) append()
Answer: d) append()
30) What is the output of the following code?
a = [1, 2, 3]
a.reverse()
print(a)
a) [3, 2, 1]
b) [1, 2, 3]
c) [3, 3, 3]
d) None of the above
Answer: a) [3, 2, 1]
31) Which of the following is used for defining a class in Python?
a) def
b) class
c) define
d) classdef
Answer: b) class
32) What is the output of the following code?
a = [1, 2, 3]
b = a
b.append(4)
print(a)
a) [1, 2, 3]
b) [1, 2, 3, 4]
c) [4, 3, 2, 1]
d) None of the above
Answer: b) [1, 2, 3, 4]
33) Which of the following is used for overriding a method in Python?
a) override
b) decorator
c) super
d) None of the above
Answer: d) None of the above
34) What is the output of the following code?
a = “Hello”
b = a.find(“l”)
print(b)
a) 2
b) 3
c) -1
d) None of the above
Answer: a) 2
35) What is the output of the following code?
a = {1, 2, 3}
b = {3, 4, 5}
print(a & b)
a) [1, 2, 3, 4, 5]
b) [1, 2]
c) [3]
d) None of the above
Answer: c) [3]
36) Which of the following is used for deleting elements from a list in Python?
a) clear()
b) delete()
c) remove()
d) pop()
Answer: c) remove()
37) What is the output of the following code?
a = [1, 2, 3, 4]
print(a[:2])
a) [1, 2]
b) [3, 4]
c) [2, 3]
d) None of the above
Answer: a) [1, 2]
38) What is the output of the following code?
a = “Hello”
b = a.split()
print(b)
a) [Hello]
b) [“Hello”]
c) [H, e, l, l, o]
d) None of the above
Answer: b) [“Hello”]
39) Which of the following is used for sorting a list in descending order in Python?
a) sort()
b) reverse()
c) sorted()
d) None of the above
Answer: b) reverse()
40) What is the output of the following code?
a = [1, 2, 3, 4]
b = [5, 6, 7, 8]
c = zip(a, b)
print(list(c))
a) [(1, 5), (2, 6), (3, 7), (4, 8)]
b) [1, 2, 3, 4, 5, 6, 7, 8]
c) Error
d) None of the above
Answer: a) [(1, 5), (2, 6), (3, 7), (4, 8)]
41) What is the output of the following code?
a = [1, 2, 3]
b = [4, 5, 6]
c = map(lambda x, y: x + y, a, b)
print(list(c))
a) [5, 7, 9]
b) [1, 2, 3, 4, 5, 6]
c) Error
d) None of the above
Answer: a) [5, 7, 9]
42) Which of the following is used for writing to a file in Python?
a) write()
b) read()
c) append()
d) open()
Answer: a) write()
43) What is the output of the following code?
a = [1, 2, 3]
b = [4, 5, 6]
c = filter(lambda x: x > 4, a + b)
print(list(c))
a) [4, 5, 6]
b) Error
c) [1, 2, 3, 4, 5, 6]
d) None of the above
Answer: a) [5, 6]
44) What is the output of the following code?
a = “Hello”
b = a[::-1]
print(b)
a) Hello
b) olleH
c) Error
d) None of the above
Answer: b) olleH
45) What is the output of the following code?
a = [1, 2, 3]
b = [4, 5, 6]
c = [x*y for x in a for y in b]
print(c)
a) [1, 2, 3, 4, 6, 8, 9, 12, 15]
b) [5, 7, 9, 11]
c) Error
d) None of the above
Answer: a) [4, 5, 6, 8, 10, 12, 12, 15, 18]
46) Which of the following is used for writing a function with an arbitrary number of arguments in Python?
a) args()
b) kwargs()
c) arbargs()
d) varyargs()
Answer: b) kwargs()
47) What is the output of the following code?
a = [1, 2, 3]
b = [x*2 for x in a if x == 2]
print(b)
a) [2]
b) Error
c) [4]
d) None of the above
Answer: c) [4]
48) What is the output of the following code?
def myfunc(n):
return lambda a : a * n
mydoubler = myfunc(2)
print(mydoubler(11))
a) 2
b) 11
c) 22
d) None of the above
Answer: c) 22
49) What is the output of the following code?
a = [1, 2, 3]
b = [1, 2, 3]
print(a == b)
print(a is b)
a) True True
b) True False
c) False True
d) False False
Answer: a) True True
50) What is the output of the following code?
a = 10
def myfunc():
global a
a = 20
myfunc()
print(a)
a) 10
b) 20
c) Error
d) None of the above