- array_change_key_case — Changes the case of all keys in an array
- array_chunk — Split an array into chunks
- array_column — Return the values from a single column in the input array
- array_combine — Creates an array by using one array for keys and another for its values
- array_count_values — Counts all the values of an array
- array_diff_assoc — Computes the difference of arrays with additional index check
- array_diff_key — Computes the difference of arrays using keys for comparison
- array_diff_uassoc — Computes the difference of arrays with additional index check which is performed by a user supplied callback function
- array_diff_ukey — Computes the difference of arrays using a callback function on the keys for comparison
- array_diff — Computes the difference of arrays
- array_fill_keys — Fill an array with values, specifying keys
- array_fill — Fill an array with values
- array_filter — Filters elements of an array using a callback function
- array_flip — Exchanges all keys with their associated values in an array
- array_intersect_assoc — Computes the intersection of arrays with additional index check
- array_intersect_key — Computes the intersection of arrays using keys for comparison
- array_intersect_uassoc — Computes the intersection of arrays with additional index check, compares indexes by a callback function
- array_intersect_ukey — Computes the intersection of arrays using a callback function on the keys for comparison
- array_intersect — Computes the intersection of arrays
- array_key_exists — Checks if the given key or index exists in the array
- array_keys — Return all the keys or a subset of the keys of an array
- array_map — Applies the callback to the elements of the given arrays
- array_merge_recursive — Merge two or more arrays recursively
- array_merge — Merge one or more arrays
- array_multisort — Sort multiple or multi-dimensional arrays
- array_pad — Pad array to the specified length with a value
- array_pop — Pop the element off the end of array
- array_product — Calculate the product of values in an array
- array_push — Push one or more elements onto the end of array
- array_rand — Pick one or more random entries out of an array
- array_reduce — Iteratively reduce the array to a single value using a callback function
- array_replace_recursive — Replaces elements from passed arrays into the first array recursively
- array_replace — Replaces elements from passed arrays into the first array
- array_reverse — Return an array with elements in reverse order
- array_search — Searches the array for a given value and returns the first corresponding key if successful
- array_shift — Shift an element off the beginning of array
- array_slice — Extract a slice of the array
- array_splice — Remove a portion of the array and replace it with something else
- array_sum — Calculate the sum of values in an array
- array_udiff_assoc — Computes the difference of arrays with additional index check, compares data by a callback function
- array_udiff_uassoc — Computes the difference of arrays with additional index check, compares data and indexes by a callback function
- array_udiff — Computes the difference of arrays by using a callback function for data comparison
- array_uintersect_assoc — Computes the intersection of arrays with additional index check, compares data by a callback function
- array_uintersect_uassoc — Computes the intersection of arrays with additional index check, compares data and indexes by separate callback functions
- array_uintersect — Computes the intersection of arrays, compares data by a callback function
- array_unique — Removes duplicate values from an array
- array_unshift — Prepend one or more elements to the beginning of an array
- array_values — Return all the values of an array
- array_walk_recursive — Apply a user function recursively to every member of an array
- array_walk — Apply a user supplied function to every member of an array
- array — Create an array
- arsort — Sort an array in reverse order and maintain index association
- asort — Sort an array and maintain index association
- compact — Create array containing variables and their values
- count — Count all elements in an array, or something in an object
- current — Return the current element in an array
- each — Return the current key and value pair from an array and advance the array cursor
- end — Set the internal pointer of an array to its last element
- extract — Import variables into the current symbol table from an array
- in_array — Checks if a value exists in an array
- key_exists — Alias of array_key_exists
- key — Fetch a key from an array
- krsort — Sort an array by key in reverse order
- ksort — Sort an array by key
- list — Assign variables as if they were an array
- natcasesort — Sort an array using a case insensitive “natural order” algorithm
- natsort — Sort an array using a “natural order” algorithm
- next — Advance the internal pointer of an array
- pos — Alias of current
- prev — Rewind the internal array pointer
- range — Create an array containing a range of elements
- reset — Set the internal pointer of an array to its first element
- rsort — Sort an array in reverse order
- shuffle — Shuffle an array
- sizeof — Alias of count
- sort — Sort an array
- uasort — Sort an array with a user-defined comparison function and maintain index association
- uksort — Sort an array by keys using a user-defined comparison function
- usort — Sort an array by values using a user-defined comparison function
Tag: array
Complete Tutorial of Multidimensional Array
Multi-dimensional arrays are arrays that instead of storing a single element, store another array at each index. To put it another way, multi-dimensional arrays should be defined as an array of arrays. Every element in this array can be an array, and they can also have additional sub-arrays within them, as the name implies. Multiple dimensions can be used to access arrays or sub-arrays in multidimensional arrays.
Dimensions:– The number of indices required to pick an element is indicated by the dimensions of a multidimensional array. To pick an element from a two-dimensional array, use two indices.
Two dimensional array:– It’s the most basic type of multidimensional array. It may be made with the help of a nested array. The index of these arrays is always a number, and they can store any sort of element. The index starts at zero by default.
Syntax:-
array (
array (elements...),
array (elements...),
...
)
Example:–
Output:-
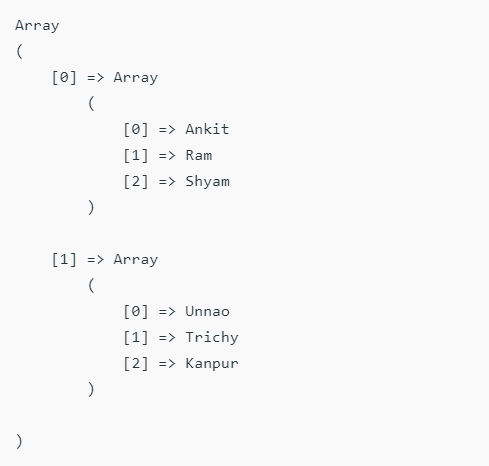
Two dimensional associative array:– Al associative arrays are similar to indexed arrays, except that instead of linear storage (indexed storage), each value can be associated with a user-defined string key.
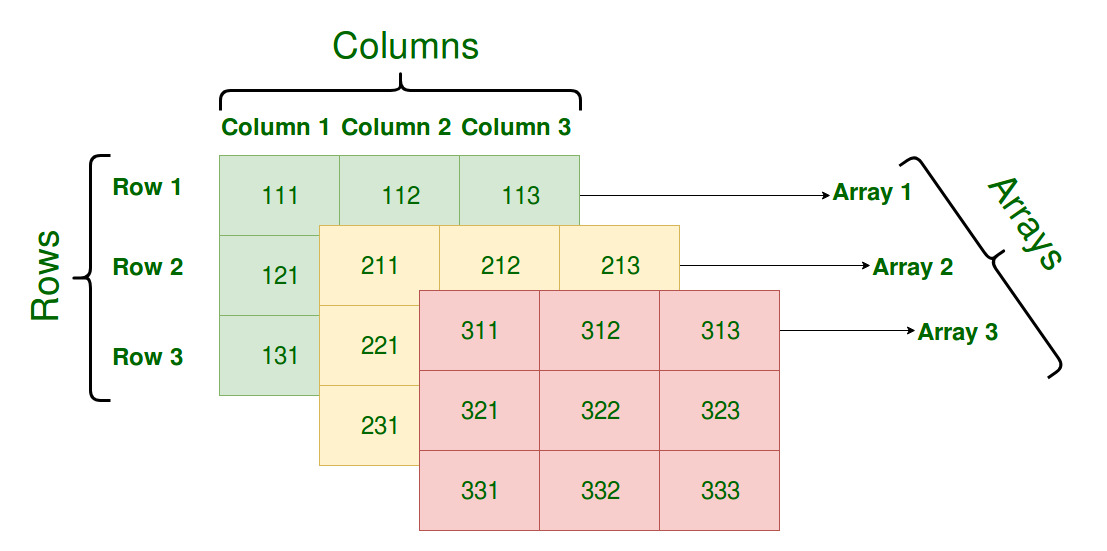
Example:–
Output:–
Display Marks:
Array
(
[Ankit] => Array
(
[C] => 95
[DCO] => 85
[FOL] => 74
)
[Ram] => Array
(
[C] => 78
[DCO] => 98
[FOL] => 46
)
[Anoop] => Array
(
[C] => 88
[DCO] => 46
[FOL] => 99
)
)
Three Dimensional Array: It is a multidimensional array in its most basic form. Three-dimensional arrays have the same initialization as two-dimensional arrays. The distinction is that as the number of dimensions grows, so does the number of nested braces.
Syntax:
array (
array (
array (elements...),
array (elements...),
...
),
array (
array (elements...),
array (elements...),
...
),
...
)
Example:
Output:
Array
(
[0] => Array
(
[0] => Array
(
[0] => 1
[1] => 2
)
[1] => Array
(
[0] => 3
[1] => 4
)
)
[1] => Array
(
[0] => Array
(
[0] => 5
[1] => 6
)
[1] => Array
(
[0] => 7
[1] => 8
)
)
)
Accessing multidimensional array elements: In PHP, there are primarily two methods for accessing multidimensional array items.
- Dimensions such as array name[‘first dimension’][‘second dimension’] can be used to retrieve elements.
- The for loop can be used to access elements.
- The for each loop may be used to access elements.
Example:
Output:

Complete Tutorial of Associative Arrays
What is Associative Arrays?
Key value pairs are stored in associative arrays. For example, a numerically indexed array might not be the ideal choice for storing a student’s marks from several subjects in an array. Instead, we might use the names of the subjects as the keys in our associative array, with their appropriate marks as the value.
Example:-
Output:–

Some Features of Associative Arrays?
Associative arrays are similar to numeric arrays in appearance, but differ in terms of index. An associative array’s index is a string that establishes a strong relationship between key and value.
For storing employee wages in an array, a numerically indexed array is not the ideal choice. Instead, you may create an associative list with the workers’ names as keys and their salary as the value.
- The variable’s name is “$variable name…”, the element’s access index number is “[‘key name’],” and the array element’s value is “value.”
- Consider the following scenario: you have a group of people and want to assign gender to each of them based on their names.
- You may achieve this by using an associative list.
- You can do so by using the code below.
Traversing the Associative Array:-
Loops may be used to go over associative arrays. The associative array can be looped through in two different ways. Using the for loop first, and then the foreach loop second.
Example:-
In this case, the array keys() function is used to identify indices with provided names, and the count() method is used to count the number of indices in associative arrays.
Output:-

Creating an associative array of mixed types:-
Output:-

Complete Tutorial of Numeric Array
What is Numeric Array?
Numeric arrays allow us to store numerous values of the same data type in a single variable rather than creating multiple variables for each value. These values can then be accessed using an index, which is always a number in numeric arrays.
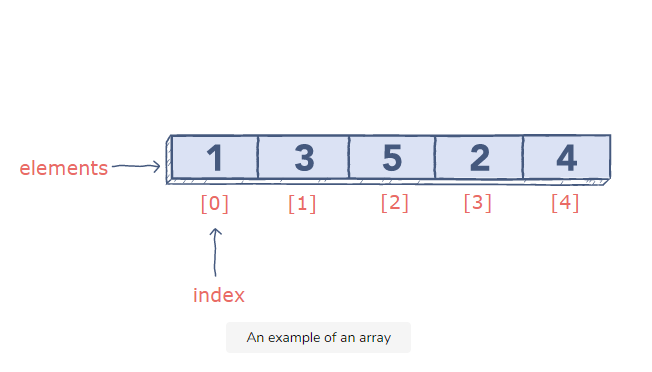
How to declare and initialize an array?
There are two ways to create an array. Let’s take a look at both these ways in the Following example:
1. Accessing the elements
You must know the index value of an array’s items in order to access them.

Example:-
2. Length of an array
The in-built function count
can be used to count the length of an array, or the number of elements in the array.

Example:-
Complete Tutorial of Arrays
What is Array?
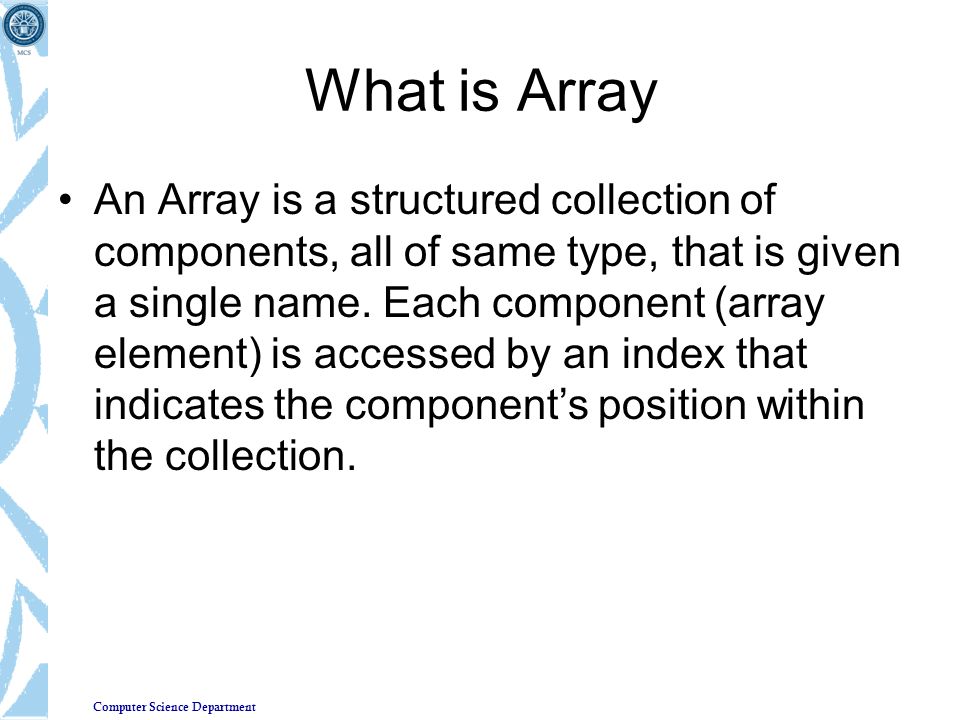
Arrays are collections of data elements with a common name. Arrays provide a framework for defining and accessing several data elements with a single identity, making data administration easier.
When dealing with several data elements, we use array.
How many types of Array?
- Numeric/Indexed Array:- In this array index will be represented by a number. By default numeric array index start from 0.
Example:-$num [0] = “ScmGalaxy”;
- Associative Array:- In this Array index/key will be represented by a string.
Example:-$fees [“ScmGalaxy”] = 500;
- Multidimensional Array:- Arrays of Arrays is known as multidimensional arrays.
Example:-$num [0] [0] = 25;
Some Array Operators are :-

How do you use in for loops in JavaScript?
for loop with Array
forEach Loop
The forEach calls a provided function once for each element in an array, in order.
Syntax: – array.forEach(function (value, index, arr) {
});
Where,
value – It is the current value of array index.
index – Array‟s index number
arr – The array object the current element belongs to
for of Loop
The for…of statement creates a loop iterating over iterable objects.
Syntax: –
for (var variable_name of array) {
}
Input from User in Array
You can get input from user in an empty array:-
- var geek= [ ];
- var geek = new Array( );
- var geek = new Array(3); // 3 is length of array
Multidimensional Array
Multidimensional array is Arrays of Arrays.
Multidimensional array can be 2D, 3D, 4D, etc.
Ex: –
2D – var name[ [ ], [ ], [ ]
Multidimensional Arra
Ajay | Dell | 10 |
Vijay | HP | 20 |
Amit | Lenovo | 30 |
[0][0] Ajay | [0][1] Dell | [2][1] 10 |
[0][0] Vijay | [1][1] HP | [2][1] 20 |
[2[0] Amit | [2][1] Zed | [2][2] 30 |
How do you use Arrays in JavaScript?
Array
Arrays are a collection of data items stored under a single name. Array provides a mechanism for declaring and accessing several data items with only one identifier, thereby simplifying the task of data management.
We use an array when we have to deal with multiple data items. Arrays are a special type of object. The typeof operator in JavaScript returns “object” for arrays.
Declaration and initialization of Array
- Using Array Literal
Syntax: – var array_name = [ ];
Declaration and initialization of Array
- Using Array Literal
Syntax: – var array_name = [value1, value2, value_n];
Declaration and initialization of Array
- Using Array Constructor
Syntax: – var array_name = new Array( );
Declaration and initialization of Array
- Using Array Constructor
This will create an empty array with 5 lengths. So this is not a good idea to use Array
Constructor if you have only a single numeric value.
Note – By default, the array starts with index 0.
Important Points
- JavaScript arrays are zero-indexed: the first element of an array is at index 0.
- Using an invalid index number returns undefined.
- It’s possible to quote the JavaScript array indexes as well (e.g., geek[‘2’] instead of geek[2]), although it’s not necessary.
- Arrays cannot use strings as element indexes but must use integers.
- There is no associative array in JavaScript. geek[“fees”] = 200;
- No advantage to use Array Constructor so better to use Array Literal for creating Arrays in JavaScript.
Accessing Array Elements
Accessing Array Elements
Access all at once
Modifying Array Elements
Removing Array Elements
Array elements can be removed using the delete operator. This operator sets the array element it is invoked on to undefined but does not change the array‟s length.
Syantx :- delete Array_name[index];
Ex:- delete dev[0];
Length Property
The length property retrieves the index of the next available position at the end of the array. The length property is automatically updated as new elements are added to the array. For this reason, length is commonly used to iterate through all elements of an array.
How do I use a default parameter in JavaScript?
Default Parameter
Syntax: –
function function_name (para1, para2=“value”, para3) // problem undefined
{
Block of statement;
}
Syntax: –
function function_name (para1, para2=“value1”, para3=“value2”)
{
Block of statement;
}
JavaScript also allows the use of arrays and null as default values.
Rest Parameters
The rest parameter allows representing an indefinite number of arguments as an array.
Syntax: –
function function_name (…args)
{
Block of statement;
}
Syntax: –
function function_name (a, …args)
{
Block of statement;
}
The rest operator must be the last parameter to a function.
Rest Vs Arguments
There are three main differences between the rest parameters and the arguments object:-
- Rest parameters are only the ones that haven’t been given a separate name, while the arguments object contains all arguments passed to the function.
- The arguments object is not a real array, while Rest Parameters are Array instances, meaning methods like sort, map, forEach or pop can be applied on it directly.
- The arguments object has additional functionality specific to itself (like the callee property).
Data types in PHP (Array)
In this tutorial we will learn about Array data type in PHP, before that you can see all the data types in PHP given below:
- String
- Integer
- Float
- Boolean
- Array
- Object
- Null
In PHP we have a special data type where we can assign number of values in single variable using array.
For example: $a = array(10,20,30);
In PHP, there are three types of arrays:
- Indexed array
- Associative array
- Multidimensional array
Indexed array:- PHP indexed array is an array which is represented by an index number by default. All elements of array are represented by an index number which starts from 0. PHP indexed array can store numbers, strings or any object. PHP indexed array is also known as numeric array.
Example:
Output:
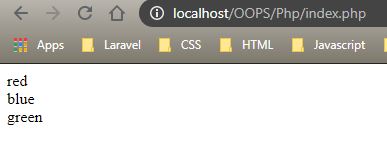
We can also use <pre> tag to print both the key and value in the output.
Output:
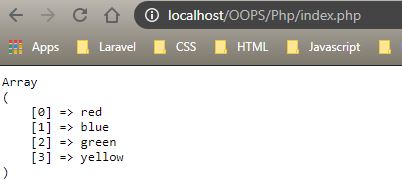
Another way to write the array in PHP which shows the same output as above:
Delete an element from array based on custom duplicate value
rajeshkumar created the topic: Delete an element from array based on custom duplicate value
Hi, I am looking for some help in one scenario.
Requirement:
Through some validation, I have strored following kinds of value in one array.
@array_name = (“Rajesh”,”Raju”,”Ram”,”John”,”peter”);
Now I know from some background that “Rajesh”, “Ram”, “peter”, is duplocated entry so i would expect my output to be:
@array_name = (“Rajesh”,”Raju”,”John”);
or
@array_name = (“Ram”,”Raju”,”John”);
or
@array_name = (“peter”,”Raju”,”John”);
I have done example program such as below but it does not satisfy me…
my $spcific_output ="";
my $output ="";
foreach my $name (@array_name)
{
if($name eq "Rajesh" || $name eq "Ram" || $name eq "peter")
{
$spcific_output = "Rajesh and Ram and peter");
}
else
{
$output .= "My Name is $name";
}
}
$output .= $spcific_output;
Any best way to achieve this????
Regards,
Rajesh Kumar
Twitt me @ twitter.com/RajeshKumarIn